1.手写单例模式
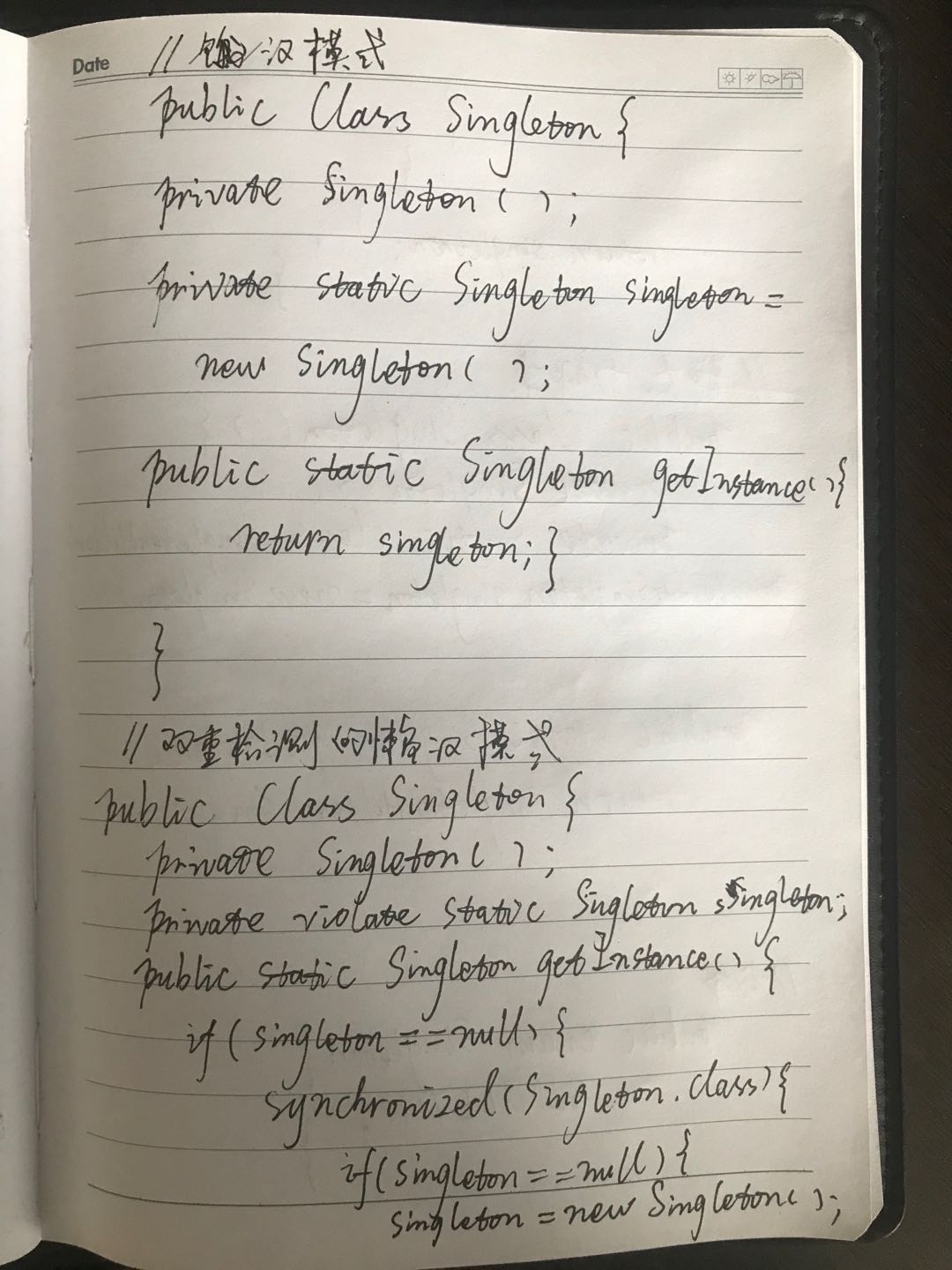
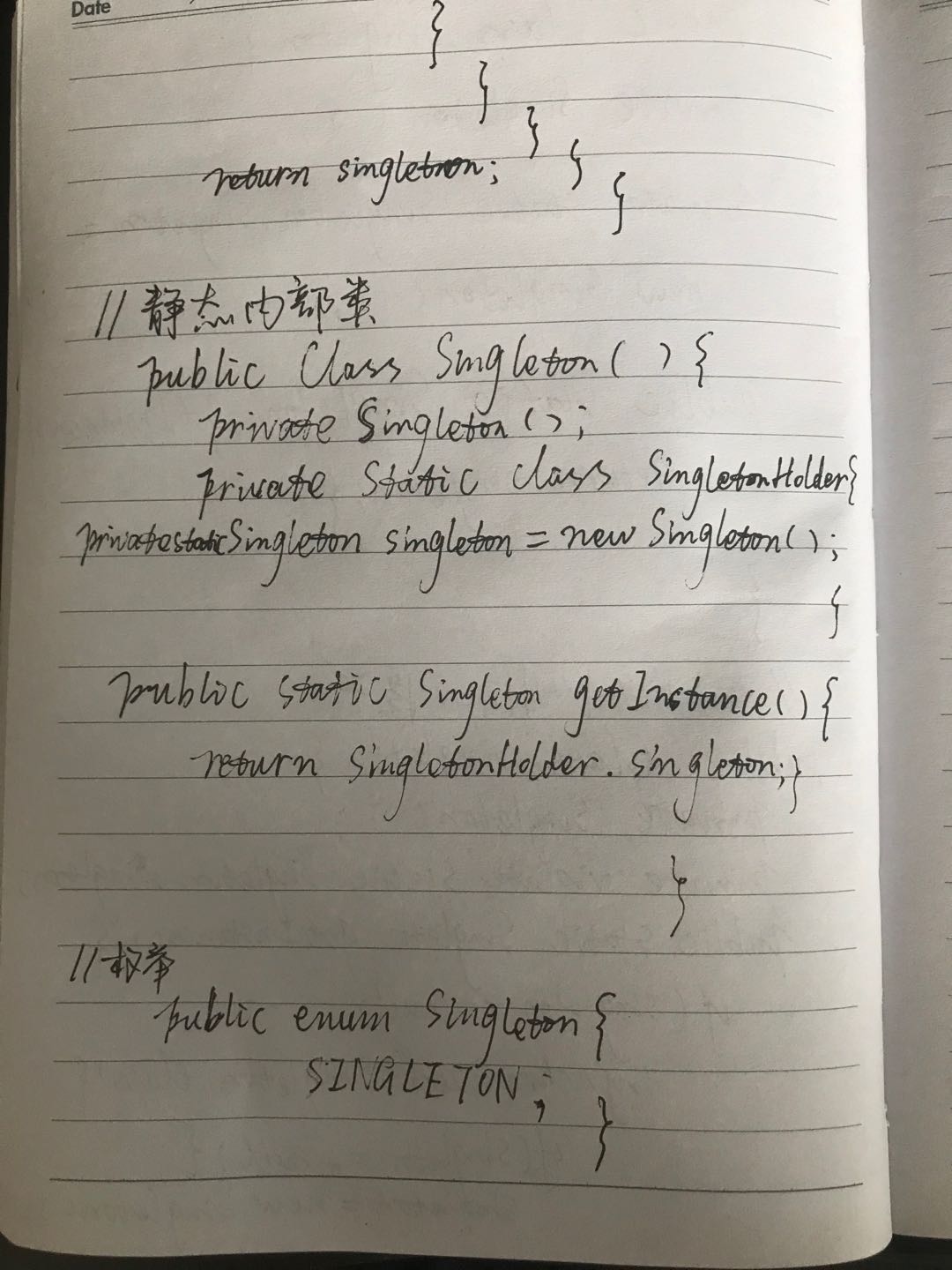
2.组合模式
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 7:21 下午
* @Version 1.0
**/
public abstract class FormNode {
private String text;
public FormNode(String text) {
this.text = text;
}
public String getText(){
return text;
}
public void draw () {
throw new UnsupportedOperationException();
}
public void add (FormNode formNode) {
throw new UnsupportedOperationException();
}
}
package com.zhk.composite;
import java.util.ArrayList;
import java.util.List;
/**
* @author zhuhk
* @create 2020-06-22 8:19 下午
* @Version 1.0
**/
public class WinForm extends FormNode {
private List<FormNode> list = new ArrayList<>();
public WinForm(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("Print WinForm(" + getText() + ")");
for (FormNode fn : list) {
fn.draw();
}
}
@Override
public void add(FormNode formNode) {
list.add(formNode);
}
}
package com.zhk.composite;
import java.util.ArrayList;
import java.util.List;
/**
* @author zhuhk
* @create 2020-06-22 8:19 下午
* @Version 1.0
**/
public class Frame extends FormNode {
private List<FormNode> list = new ArrayList<>();
public Frame(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("Print Frame(" + getText() + ")");
for (FormNode fn : list) {
fn.draw();
}
}
@Override
public void add(FormNode formNode) {
list.add(formNode);
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class Button extends FormNode {
public Button(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print Button(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class CheckBox extends FormNode {
public CheckBox(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print CheckBox(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class Lable extends FormNode {
public Lable(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print Lable(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class LinkLable extends FormNode {
public LinkLable(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print LinkLable(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class PasswordBox extends FormNode {
public PasswordBox(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print PasswordBox(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class Picture extends FormNode {
public Picture(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print Picture(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-22 9:44 下午
* @Version 1.0
**/
public class TextBox extends FormNode {
public TextBox(String text) {
super(text);
}
@Override
public void draw() {
System.out.println("print TextBox(" + getText() + ")");
}
}
package com.zhk.composite;
/**
* @author zhuhk
* @create 2020-06-23 11:28 上午
* @Version 1.0
**/
public class Client {
public static void main(String[] args) {
WinForm winForm = new WinForm("WINDOWS窗口");
Picture picture = new Picture("LOGO图片");
Button button = new Button("登录");
Button button1 = new Button("注册");
Frame frame = new Frame("FRAME1");
Lable lable = new Lable("用户名");
TextBox textBox = new TextBox("文本框");
Lable lable1 = new Lable("密码");
PasswordBox passwordBox = new PasswordBox("密码框");
CheckBox checkBox = new CheckBox("复选框");
TextBox textBox1 = new TextBox("记住用户名");
LinkLable linkLable = new LinkLable("忘记密码");
winForm.add(picture);
winForm.add(button);
winForm.add(button1);
winForm.add(frame);
frame.add(lable);
frame.add(textBox);
frame.add(lable1);
frame.add(passwordBox);
frame.add(checkBox);
frame.add(textBox1);
frame.add(linkLable);
winForm.draw();
}
}
运行结果如下:
Connected to the target VM, address: '127.0.0.1:51600', transport: 'socket'
Print WinForm(WINDOWS窗口)
print Picture(LOGO图片)
print Button(登录)
print Button(注册)
Print Frame(FRAME1)
print Lable(用户名)
print TextBox(文本框)
print Lable(密码)
print PasswordBox(密码框)
print CheckBox(复选框)
print TextBox(记住用户名)
print LinkLable(忘记密码)
Disconnected from the target VM, address: '127.0.0.1:51600', transport: 'socket'